So my default verbose and debug messages might not really be good looking, so I needed to standardize how I wrote them. I wanted the time, function name and message to be printed and standardized. So I came up with this invoke way.
How do I execute a script block from a variable?
Well first we need to save the standard to a variable and then execute it when needed. Well that is easy in powershell.
$command = {Get-Date}
&$command
$command
variable and then using the invoke operator runs it. If you run the later command again you will notice that the Get-Date is executed now to.
Building it from scratch
But we wanted to add the function name too. So lets look into that. I wrote an article before that talked about good constants in Powershell. These aren’t really constants but Powershell variables that powershell itself populates. Tada if you look in $MyInvocation.MyCommand.Name you will find the current functionname. So lets try that on the commandline:
function Test-VirotFunction {
PS C:\> $MyInvocation.MyCommand.Name
PS C:\>
function Test-VirotFunction {
"This used to be my playground $($MyInvocation.MyCommand.Name)"
}
Test-VirotFunction
PS C:\> $message = {param($string)"You sent: $string"}
PS C:\> &$message 'Hello'
You sent: Hello
A pot hole
Function Test-VirotMessages{
[CmdletBinding()]
Param()
Process
{
$DebugMessage = {Param([string]$Message);"$(get-date -Format 's') [$($MyInvocation.MyCommand.Name)]: $Message"}
Write-Verbose (&$DebugMessage 'Testing Verbose message')
}
}
Test-VirotMessages -Verbose
Now all at once
Function Test-VirotMessages{
[CmdletBinding()]
Param()
Begin
{
$DebugMessage = {Param([string]$Message);"$(get-date -Format 's') [$((Get-Variable -Scope 1 MyInvocation -ValueOnly).MyCommand.Name)]: $Message"}
}
Process
{
Write-Debug (&$DebugMessage 'Test Debug message')
Write-Verbose (&$DebugMessage 'Testing Verbose message')
}
}
Test-VirotMessages -Verbose
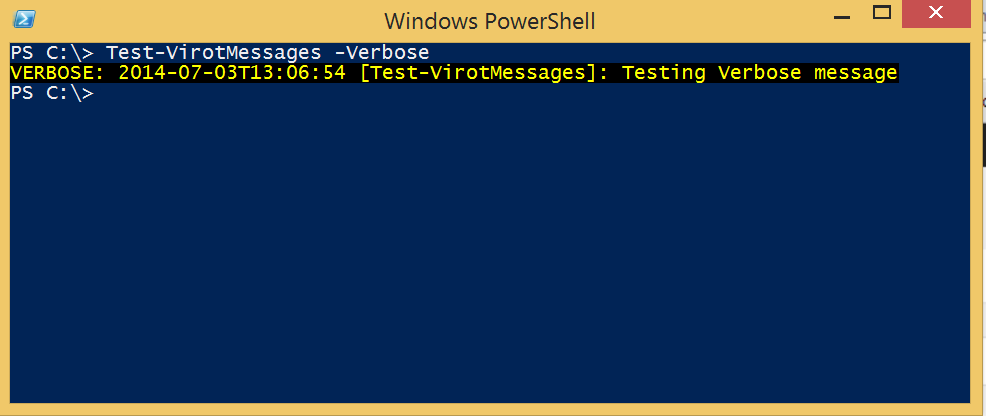